The WordPress ecosystem now hosts a range of fully developed email solutions provided by numerous plugins. Two of the most prominent examples are WP Mail SMTP and Easy WP SMTP. While I have no particular objections to these plugins, I believe that for experienced developers, they can be overly complex. Most of their features extend far beyond the typical use cases that developers actually need.
The most common requirement for most developers is simply having the ability to send emails over SMTP. Fortunately, WordPress already comes equipped with PHPMailer, which makes this task much easier.
You don’t necessarily need a dedicated plugin to connect to a third-party SMTP provider. Instead, you can directly configure your SMTP credentials—such as those from Amazon SES—within your plugin or theme code.
Once you’ve created and logged into your AWS account, navigate to the Amazon SES console. There, you should see a screen similar to the one below:
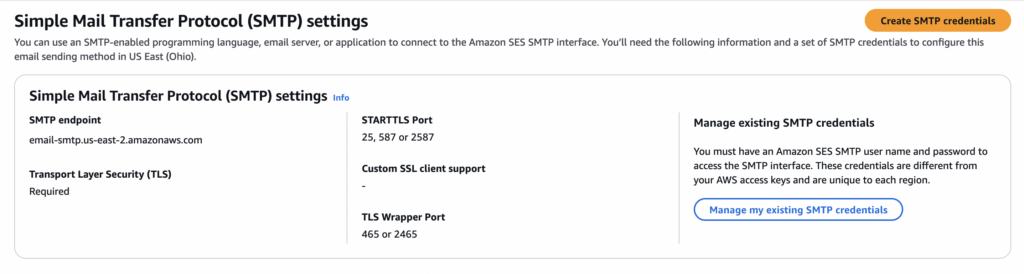
If not done already, create new SMTP credentials by clicking the button “Create SMTP Credentials” and follow their instructions. It’s important to note that AWS uses a special type of user for SMTP authentication—regular IAM user credentials won’t work for sending emails. After completing this process, you’ll have the necessary SMTP security credentials for integration.
Set your credentials in wp-config.php
as follows:
define( 'SMTP_HOST', 'email-smtp.us-east-2.amazonaws.com' ); // <- Change to your SMTP endpoint.
define( 'SMTP_PORT', 587 );
define( 'SMTP_AUTH', true );
define( 'SMTP_USERNAME', 'AKIAIOSFODNN7EXAMPLE' ); // <- Change to access key id.
define( 'SMTP_PASSWORD', 'wJalrXUtnFEMI/K7MDENG/bPxRfiCYEXAMPLEKEY' ); // <- Change to your secret access key.
define( 'SMTP_SECURE', 'tls' );
Then, add following code to the phpmailer_ini hook to your theme or plugin code.
// Ensure constants are defined (in case wp-config not updated)
if (defined('SMTP_HOST') && defined('SMTP_PORT') && defined('SMTP_USERNAME') && defined('SMTP_PASSWORD')) {
function on_smtp_configure($mail)
{
$mail->isSMTP();
$mail->Host = SMTP_HOST;
$mail->SMTPAuth = SMTP_AUTH;
$mail->Port = SMTP_PORT;
$mail->Username = SMTP_USERNAME;
$mail->Password = SMTP_PASSWORD;
$mail->SMTPSecure = SMTP_SECURE;
}
add_action('phpmailer_init', __NAMESPACE__ . '\on_smtp_configure');
}
Enjoy!